Using Locales – Localization
By Gigi Rosen / August 24, 2023 / No Comments / Compact Number Parsing, Oracle Certifications, The ZonedDateTime Class
18.1 Using Locales
A locale represents a specific geographical, political, or cultural region. Its two most important attributes are language and country. Certain classes in the Java standard library provide locale-sensitive operations. For example, they provide methods to format values that represent dates, currencies, and numbers according to a specific locale. Adapting a program to a specific locale is called localization.
A locale is represented by an instance of the class java.util.Locale. A locale object can be created using the following constructors:
Locale(String language)
Locale(String language, String country)
The language string is normalized to lowercase and the country string to upper-case. The language argument is an ISO-639 Language Code (which uses two lowercase letters), and the country argument is an ISO-3166 Country Code (which uses two uppercase letters) or a UN M.49 three-digit area code— although the constructors do not impose any constraint on their length or perform any syntactic checks on the arguments.
For the one-argument constructor, the country remains undefined.
Examples of selected language codes and country codes are given in Table 18.1 and Table 18.2, respectively.
Table 18.1 Selected Language Codes
Language code | Language |
“en” | English |
“no” | Norwegian |
“fr” | French |
Table 18.2 Selected Country/Region Codes
Country/Region code | Country/Region |
“US” | United States (U.S.) |
“GB” | Great Britain (GB) |
“NO” | Norway |
“FR” | France |
“003” | North America |
The Locale class also has predefined locales for certain languages, irrespective of the region where they are spoken, as shown in Table 18.3.
Table 18.3 Selected Predefined Locales for Languages
Constant | Language |
Locale.ENGLISH | Locale with English (new Locale(“en”)) |
Locale.FRENCH | Locale with French (new Locale(“fr”)) |
Locale.GERMAN | Locale with German (new Locale(“de”))—that is, Deutsch |
The Locale class also has predefined locales for certain combinations of countries and languages, as shown in Table 18.4.
Table 18.4 Selected Predefined Locales for Countries
Constant | Country |
Locale.US | Locale for U.S. (new Locale(“en”,”US”)) |
Locale.UK | Locale for United Kingdom/Great Britain (new Locale(“en”,”GB”)) |
Locale.CANADA_FRENCH | Locale for Canada with French language (new Locale(“fr”,”CA”)) |
Normally a program uses the default locale on the platform to provide localization. The Locale class provides a get and a set method to manipulate the default locale.
static Locale getDefault()
static void setDefault(Locale newLocale)
The first method returns the default locale, and the second one sets a specific locale as the default locale.
static Locale[] getAvailableLocales()
Returns an array of all installed locales.
static Locale forLanguageTag(String languageTag)
Returns a locale for the specified IETF BCP 47 language tag string, which allows extended locale properties, such as a calendar or a numeric system. An example would be creating a locale based on the language tag “zh-cmn-Hans-CN”, which stands for Mandarin Chinese, Simplified script, as used in China. Note that the locale qualifiers (also called subtags) in the language tag are separated by hyphens (-).
String getCountry()
Returns the country/region code for this locale, or null if the code is not defined for this locale.
String getLanguage()
Returns the language code of this locale, or null if the code is not defined for this locale.
String getDisplayCountry()
String getDisplayCountry(Locale inLocale)
Returns a name for the locale’s country that is appropriate for display, depending on the default locale in the first method or the inLocale argument in the second method.
String getDisplayLanguage()
String getDisplayLanguage(Locale inLocale)
Return a name for the locale’s language that is appropriate for display, depending on the default locale in the first method or the inLocale argument in the second method.
String getDisplayName()
String getDisplayName(Locale inLocale)
Return a name for the locale that is appropriate for display.
String toString()
Returns a text representation of this locale in the format “languageCode_countryCode”. Language is always lowercase and country is always uppercase. If either of the codes is not specified in the locale, the _ (underscore) is omitted.
A locale is an immutable object, having two sets of get methods to return the display name of the country and the language in the locale. The first set returns the display name of the current locale according to the default locale, while the second set returns the display name of the current locale according to the locale specified as an argument in the method call.
Methods that require a locale for their operation are called locale-sensitive. Methods for formatting numbers, currencies, dates, and the like use the locale information to determine how such values should be formatted. A locale does not provide such services. Subsequent sections in this chapter provide examples of locale-sensitive classes (Figure 18.1, p. 1115).
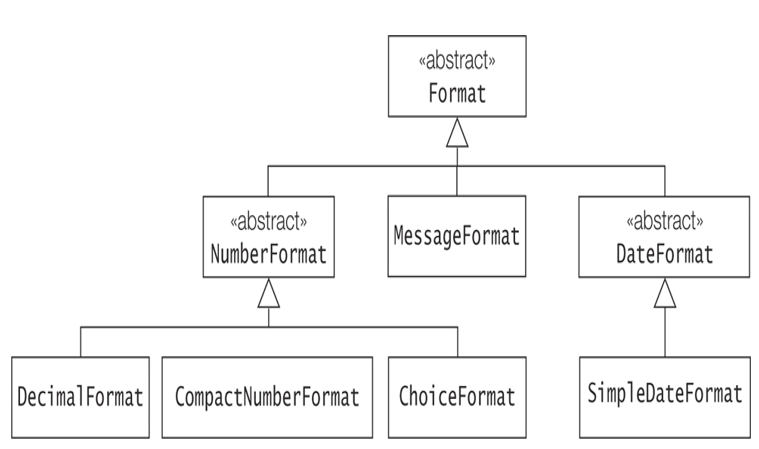
Figure 18.1 Core Classes for Formatting in the java.text Package
Example 18.1 illustrates the use of the get methods in the Locale class. The call to the getDefault() method at (1) returns the default locale. The method call locNO.getDisplayCountry() returns the country display name (Norwegian) of the Norwegian locale according to the default locale (which in this case is United States), whereas the method call locNO.getDisplayCountry(locFR) returns the country display name (Norvège) of the Norwegian locale according to the French locale.
Example 18.1 also illustrates that an application can change its default locale pro-grammatically at any time. The call to the setDefault() method at (2) sets the default locale to that of Germany. The name of the Norwegian locale is displayed according to this new default locale.
Example 18.1 Understanding Locales
import java.util.Locale;
public class LocalesEverywhere {
public static void main(String[] args) {
Locale locDF = Locale.getDefault(); // (1)
Locale locNO = new Locale(“no”, “NO”); // Locale: Norwegian/Norway
Locale locFR = new Locale(“fr”, “FR”); // Locale: French/France
System.out.println(“Default locale is: ” + locDF.getDisplayName());
System.out.println(“Display country (language) for Norwegian locale:”);
System.out.printf(“In %s: %s (%s)%n”, locDF.getDisplayCountry(),
locNO.getDisplayCountry(locDF), locNO.getDisplayLanguage(locDF));
System.out.printf(“In %s: %s (%s)%n”, locNO.getDisplayCountry(),
locNO.getDisplayCountry(locNO), locNO.getDisplayLanguage(locNO));
System.out.printf(“In %s: %s (%s)%n”, locFR.getDisplayCountry(),
locNO.getDisplayCountry(locFR), locNO.getDisplayLanguage(locFR));
System.out.println(“\nChanging the default locale.”);
Locale.setDefault(Locale.GERMANY); // (2) Locale: German/Germany
locDF = Locale.getDefault();
System.out.println(“Default locale is: ” + locDF.getDisplayName());
System.out.printf(“Interpreting %s locale information in %s locale.%n”,
locNO.getDisplayName(), locDF.getDisplayName());
}
}
Output from the program:
Default locale is: English (United States)
Display country (language) for Norwegian locale:
In United States: Norway (Norwegian)
In Norway: Norge (norsk)
In France: Norvège (norvégien)
Changing the default locale.
Default locale is: Deutsch (Deutschland)
Interpreting Norwegisch (Norwegen) locale information in Deutsch (Deutschland)
locale.